Unleashing the Power of Caching in Rails
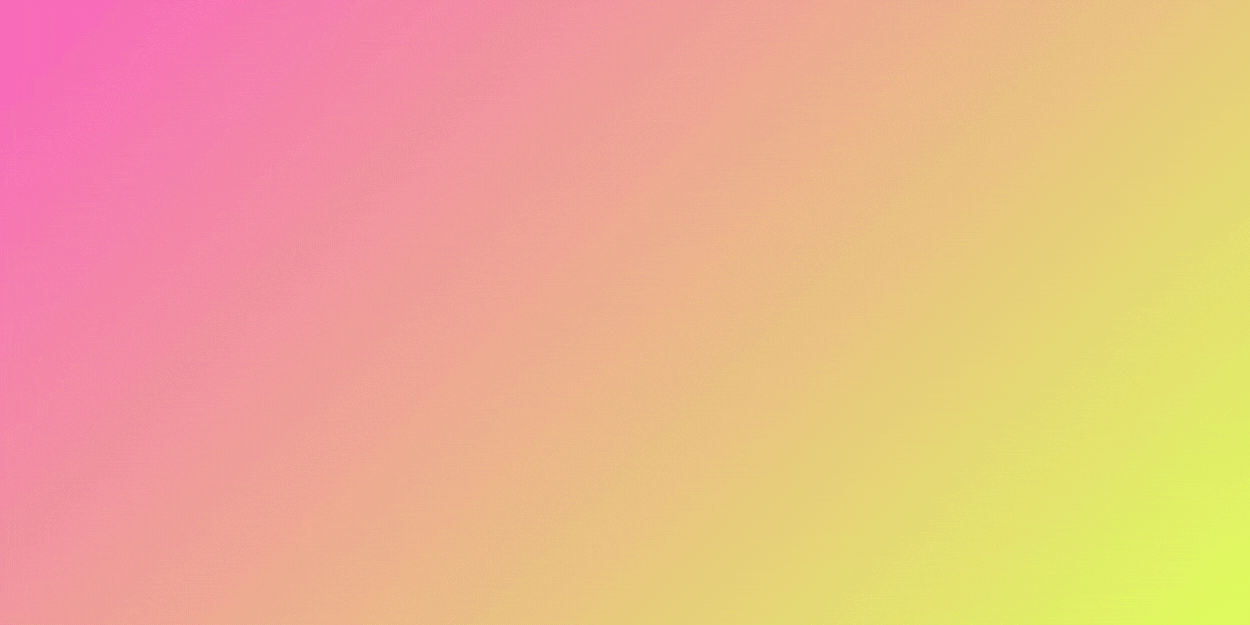
In the fast-paced world of web development, where every millisecond counts, optimizing the performance of your Rails application is crucial. One powerful technique to achieve this is caching. Caching helps reduce database queries, server load, and overall response times by storing and reusing previously computed or fetched data. In this blog post, we'll delve into the world of caching in Rails, exploring various caching strategies, tools, and best practices to supercharge your application.
Understanding Caching in Rails
1. Page Caching
Page caching is the simplest form of caching. It involves storing the entire HTML output of a page and serving it directly for subsequent requests. This is highly effective for static pages that don't change frequently.
To enable page caching in Rails, use the caches_page
method in your controller:
class HomeController < ApplicationController
caches_page :index
end
This will create a cached HTML file for the index
action in the public
directory.
2. Action Caching
Similar to page caching, action caching stores the output of a controller action but allows for dynamic content. It's beneficial for actions that have some variability but still don't change often.
class ProductsController < ApplicationController
caches_action :show, expires_in: 1.hour
end
The expires_in
option sets the time until the cache expires, and the action is re-executed.
3. Fragment Caching
For more fine-grained control, fragment caching allows you to cache specific parts of a view. This is useful when only a portion of a page is dynamic.
<% cache @product do %>
<!-- dynamic content here -->
<% end %>
In this example, the content inside the cache
block will only be computed and stored if the @product
object has changed.
Cache Stores
Rails supports multiple cache stores, including :memory_store
, :file_store
, :redis_store
, and more. Choose the one that best fits your application's needs and infrastructure.
# config/environments/production.rb
config.cache_store = :redis_store, 'redis://localhost:6379/0/cache', { expires_in: 90.minutes }
Key-based Cache Expiration
Explicitly managing cache expiration is crucial to ensure your users always see up-to-date content. Use unique cache keys and sweepers to expire caches when relevant data changes.
# Using a model's timestamp as part of the cache key
<% cache @product, @product.updated_at do %>
<!-- dynamic content here -->
<% end %>
# In the model
class Product < ApplicationRecord
after_save { |product| product.touch }
end
Sweeping Caches
Cache sweepers automatically expire caches when related data changes. For example, if you have a blog and want to expire the cache for the home page when a new post is created:
# app/models/post_observer.rb
class PostObserver < ActiveRecord::Observer
def after_save(post)
expire_cache_for_home_page
end
private
def expire_cache_for_home_page
expire_page(controller: 'home', action: 'index')
end
end
Conclusion
Caching is a powerful tool to enhance the performance and responsiveness of your Rails application. By employing various caching strategies, choosing the right cache store, and managing cache expiration effectively, you can significantly boost your application's speed and deliver a seamless user experience. Experiment with different caching techniques, monitor your application's performance, and continually optimize to keep up with the evolving needs of your users. Happy caching!