Rails Indexing for Performance Improvement.
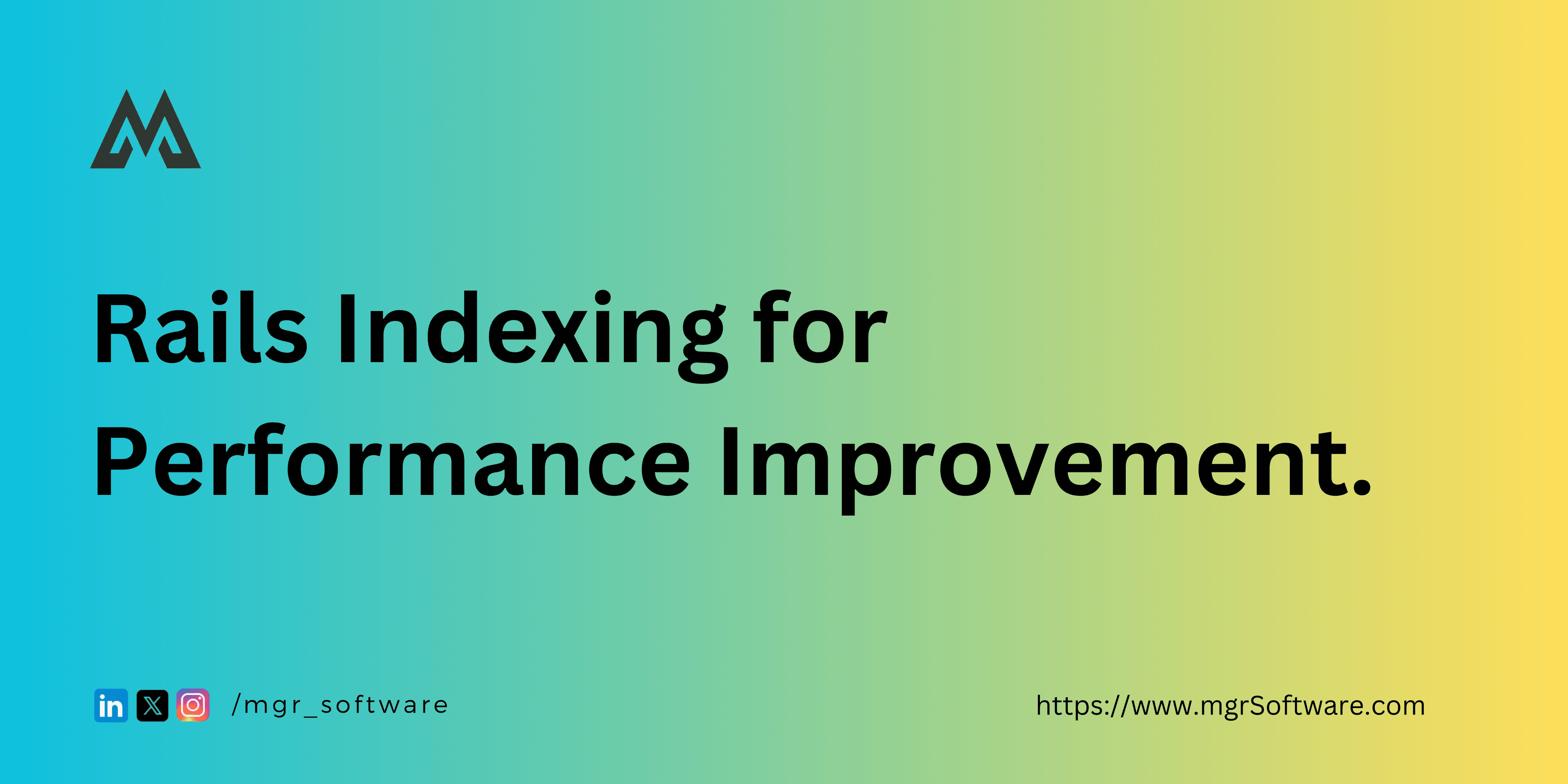
In the world of web development, speed and efficiency are paramount. When building a Ruby on Rails application, optimizing database performance is a critical aspect of ensuring your web application runs smoothly and provides a great user experience. One of the most effective ways to boost database performance is by using indexing. In this blog post, we'll look into the world of Rails indexing and explore how it can significantly improve the performance of your application.
What is Indexing?
An index is a database structure that enhances the speed of data retrieval operations on a database table. Indexes are created on one or more columns of a table, and they store a sorted copy of the data values in those columns. Think of it as an organized roadmap to your data, allowing the database management system to locate records quickly without scanning the entire table.
Why Use Indexes in Rails?
When you query a database, especially one with a large dataset, the database management system must sift through a lot of data to find the relevant information. Without proper indexes, these searches can become painfully slow. By creating appropriate indexes, you can dramatically reduce the time it takes to fetch data, improving the overall performance of your Rails application.
How to Create Indexes in Rails
Rails provides a simple and intuitive way to add indexes to your database tables. Here's how you can create indexes for your Rails models:
-
Migration Files: Indexes are typically added through migration files. You can create a new migration to add an index or update an existing table with an index. For instance, if you want to add an index to the "email" column in a "users" table, you would run:
rails generate migration AddIndexToUsersEmail
-
Define the Index in the Migration: Open the generated migration file and add the index definition using the
add_index
method:class AddIndexToUsersEmail < ActiveRecord::Migration[6.0] def change add_index :users, :email, unique: true end end
In this example, we are adding a unique index to the "email" column of the "users" table. The
unique: true
option ensures that each email in the table is unique. -
Run the Migration: To apply the index to the database, run:
rails db:migrate
Types of Indexes
Rails supports several types of indexes, each suitable for different use cases. Here are a few common types:
-
Single-Column Index: As shown in the example above, this index type is created on a single column.
-
Composite Index: This type involves indexing multiple columns together. It's useful when you often query data based on the combination of these columns.
-
Unique Index: Ensures that values in the indexed column(s) are unique, preventing duplicate data.
-
Partial Index: Allows you to index a subset of rows in a table based on a condition. This can be useful for optimizing specific queries.
Best Practices for Rails Indexing
-
Identify Bottlenecks: Start by identifying the queries that are slow. Use tools like the Rails console, query logs, and profiling to pinpoint performance bottlenecks.
-
Keep Indexes Simple: Avoid creating too many indexes on a single table, as it can lead to performance issues during insert, update, or delete operations.
-
Regularly Maintain Indexes: Indexes can become fragmented over time. Consider rebuilding or reorganizing them periodically to maintain optimal performance.
-
Monitor Performance: Continuously monitor the performance of your database to detect and address issues early.
-
Understand Query Execution Plans: Familiarize yourself with the query execution plans generated by your database. This can help you fine-tune your indexing strategy.
-
Test Thoroughly: Before applying indexes to your production database, test them in a development environment to ensure they improve performance as expected.
Conclusion
In the world of Ruby on Rails, proper indexing is a vital tool for improving the performance of your application. With the right indexes in place, you can dramatically speed up data retrieval and provide a seamless user experience. Take the time to analyze your application's needs, create well-considered indexes, and continuously monitor and optimize your database for optimal performance. Rails indexing is a powerful technique that can make a significant difference in your web application's speed and efficiency.