Optimizing Performance in React Applications
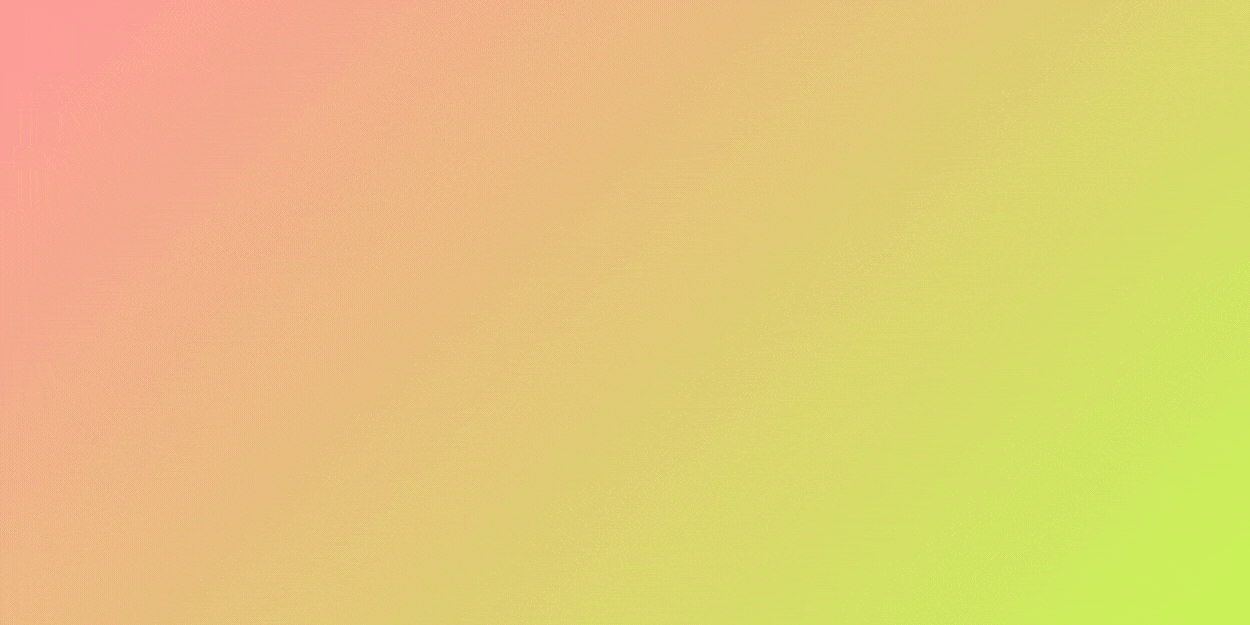
In the fast-paced world of web development, user experience is paramount, and application performance plays a crucial role in ensuring a seamless interaction. React, being a popular JavaScript library for building user interfaces, offers various tools and techniques to optimize the performance of your applications. In this blog post, we'll explore key performance optimization techniques in React, ranging from memoization and PureComponent to React.memo, and the useMemo and useCallback hooks. Additionally, we'll delve into the usage of React DevTools for profiling and optimizing components.
Understanding React Performance Optimization Techniques
1. Memoization:
Memoization is a powerful optimization technique that involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. In React, memoization can be implemented using the useMemo
hook, allowing you to memoize the result of a computation and only recompute it when the dependencies change.
Example:
const MemoizedComponent = React.memo(({ data }) => {
// Memoized computation using useMemo
const processedData = React.useMemo(() => processData(data), [data]);
return <div>{processedData}</div>;
});
2. PureComponent:
PureComponent is a class component in React that comes with a built-in implementation of shouldComponentUpdate
. It performs a shallow comparison of the current and next props and state, preventing unnecessary renders if the data hasn't changed.
Example:
class MyPureComponent extends React.PureComponent {
render() {
return <div>{this.props.data}</div>;
}
}
3. React.memo:
The React.memo
higher-order component is used for memoizing functional components. It works similarly to PureComponent
by preventing re-renders if the props remain the same.
Example:
const MemoizedComponent = React.memo(({ data }) => {
return <div>{data}</div>;
});
4. useMemo and useCallback Hooks:
The useMemo
and useCallback
hooks are essential for memoizing values and functions, respectively. They ensure that expensive calculations or functions are only recomputed when necessary.
Example:
const MemoizedComponent = ({ fetchData }) => {
const memoizedCallback = React.useCallback(() => fetchData(), [fetchData]);
return <button onClick={memoizedCallback}>Fetch Data</button>;
};
Profiling and Optimizing with React DevTools
In addition to these in-code optimization techniques, React DevTools provides a powerful set of tools for profiling and optimizing your components.
-
Profiling Mode: React DevTools offers a Profiler tab that allows you to profile your components and identify performance bottlenecks. Use it to visualize component render times and understand which components are causing re-renders.
-
Highlight Updates: Enabling the "Highlight Updates" feature in React DevTools visually highlights components that are re-rendering. This helps identify unnecessary renders and optimize your component tree.
-
React.memo and useMemo Insights: React DevTools provides insights into components wrapped with
React.memo
and the usage ofuseMemo
. It indicates when memoization is effective and helps you ensure that your optimizations are working as intended. -
Component Tree Visualization: The Component Tree view in React DevTools lets you inspect the hierarchy of your components and their relationships. Use this view to identify areas where you can apply memoization and optimize the component tree structure.
Conclusion:
Optimizing performance in React applications is a continuous process that involves a combination of in-code techniques and the use of tools like React DevTools. By incorporating memoization, using PureComponent, React.memo, and the useMemo and useCallback hooks, you can significantly enhance the efficiency of your React applications. Additionally, React DevTools provides invaluable insights into your application's performance, aiding in the identification of bottlenecks and the optimization of your component tree.
Remember, while performance optimization is crucial, it's equally important to strike a balance and avoid premature optimization. Profile your application, identify the critical paths, and optimize strategically to provide users with a smooth and responsive experience.