Unveiling the Magic of React Memoization: A Simple Guide
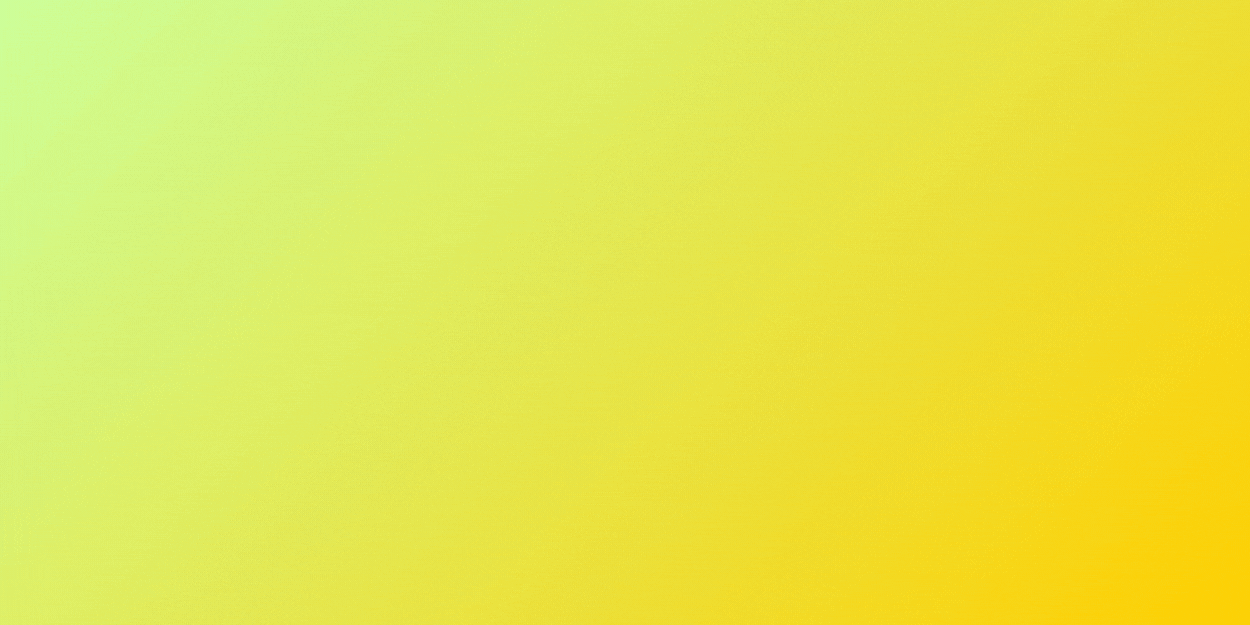
Introduction:
Welcome to the fascinating world of React Memoization, a powerful technique that can supercharge the performance of your React applications. In this blog post, we'll embark on a journey to demystify memoization, understand its significance, and explore how it can optimize the rendering process in React components.
Understanding React Rendering:
Before diving into memoization, let's briefly recap how React handles rendering. React components re-render whenever their state or props change. This dynamic nature is what makes React highly reactive, enabling the creation of dynamic and interactive user interfaces.
The Need for Optimization:
While React's reactivity is fantastic, it can lead to unnecessary re-renders. Consider a scenario where a component re-renders even though its state or props remain unchanged. This inefficiency can result in performance bottlenecks, especially in large applications.
Enter React Memoization:
React Memoization is a technique designed to address the issue of unnecessary re-renders by optimizing the rendering process. Memoization involves caching the results of a function and returning the cached result when the same inputs occur again. In React, this translates to preventing the re-execution of a component's render function if its inputs (props and state) remain the same.
How to Use Memoization in React:
React provides a built-in method called React.memo to implement memoization effortlessly. Here's a quick guide on how to use it:
Copy code
import React from 'react';
const MyComponent = React.memo((props) => {
// Component logic here
});
export default MyComponent;
By wrapping our component with React.memo, React will automatically memoize it, preventing unnecessary re-renders when the component receives the same props.
Benefits of React Memoization:
-
Performance Boost:
Memoization significantly reduces unnecessary re-renders, leading to improved performance and a smoother user experience. -
Optimized Rendering:
Components wrapped with React.memo only re-render when their props or state change, ensuring that the rendering process is efficient and focused. -
Simplified Code:
Implementing memoization is a simple yet powerful way to optimize components without introducing complex code changes.
Conclusion:
In conclusion, React Memoization is a game-changer for optimizing the rendering process in React applications. By intelligently caching component results, we can ensure that our applications remain performant and responsive. Consider integrating memoization into your React components to unlock a new level of efficiency and responsiveness in your web applications. Happy coding!